C++ Programming
1 Mohannad Al-Kubaisi
INTRODUCTION
A computer is a useful tool for solving a great variety of problems. To make a
computer do anything (i.e. solve a problem), you have to write a computer
program. In a computer program you tell a computer, step by step, exactly what
you want it to do. The computer then executes the program, following each step
mechanically, to accomplish the end goal.
Algorithm and flowchart are the powerful tools for learning programming. An
algorithm is a step-by-step analysis of the process, while a flowchart explains the
steps of a program in a graphical way. Algorithm and flowcharts helps to clarify all
the steps for solving the problem.
ALGORITHMS
The word “algorithm” relates to the name of the mathematician Al-khowarizmi,
which means a procedure or a technique. Software Engineer commonly uses an
algorithm for planning and solving the problems. An algorithm is a sequence of
steps to solve a particular problem or algorithm is an ordered set of unambiguous
steps that produces a result and terminates in a finite time.
Algorithm has the following characteristics
Input: An algorithm may or may not require input.
Output: Each algorithm is expected to produce at least one result.
Definiteness: Each instruction must be clear and unambiguous.
Finiteness: If the instructions of an algorithm are executed, the algorithm
should terminate after finite number of steps.
The algorithm and flowchart include following three types of control structures.
1. Sequence: In the sequence structure, statements are placed one after the
other and the execution takes place starting from up to down.
2. Branching (Selection): In branch control, there is a condition and according
to a condition, a decision of either TRUE or FALSE is achieved. Generally, the
‘IF-THEN’ is used to represent branch control.
3. Loop (Repetition): The Loop or Repetition allows a statement(s) to be
executed repeatedly based on certain loop condition e.g. WHILE, FOR loops.
C++ Programming
2 Mohannad Al-Kubaisi
HOW TO WRITE ALGORITHMS
Step 1: Define your algorithms input: Many algorithms take in data to be
processed, e.g. to calculate the area of rectangle input may be the rectangle height
and rectangle width.
Step 2: Define the variables: Algorithm's variables allow you to use it for more than
one place. We can define two variables for rectangle height and rectangle width as
HEIGHT and WIDTH (or H & W). We should use meaningful variable name.
Step 3: Outline the algorithm's operations: Use input variable for computation
purpose, e.g. to find area of rectangle multiply the HEIGHT and WIDTH variable and
store the value in new variable (say) AREA. An algorithm's operations can take the
form of multiple steps and even branch, depending on the value of the input
variables.
Step 4: Output the results of your algorithm's operations: In case of area of
rectangle output will be the value stored in variable AREA. if the input variables
described a rectangle with a HEIGHT of 2 and a WIDTH of 3, the algorithm would
output the value of 6.
FLOWCHART
The first design of flowchart goes back to 1945 which was designed by John Von
Neumann. Unlike an algorithm, Flowchart uses different symbols to design a
solution to a problem. It is another commonly used programming tool. By looking
at a Flowchart can understand the operations and sequence of operations
performed in a system. Flowchart is often considered as a blueprint of a design
used for solving a specific problem.
Flowchart is diagrammatic /Graphical representation of sequence of steps to solve
a problem. To draw a flowchart following standard symbols are use.
C++ Programming
3 Mohannad Al-Kubaisi
The language used to write algorithm is simple and similar to day-to-day life
language. The variable names are used to store the values. The value store in
variable can change in the solution steps. In addition some special symbols are used
as below.
C++ Programming
4 Mohannad Al-Kubaisi
Assignment Symbol (← or =) is used to assign value to the variable.
e.g. to assign value 5 to the variable HEIGHT, statement is
HEIGHT ← 5 or HEIGHT = 5
The symbol ‘=’ is used in most of the programming language as an assignment
symbol, the same has been used in all the algorithms and flowcharts in the manual.
The statement C = A + B means that add the value stored in variable A and variable
B then assign/store the value in variable C.
The statement R = R + 1 means that add I to the value stored in variable R and then
assign/store the new value in variable R, in other words increase the value of
variable R by 1
Mathematical Operators
Relational Operators
C++ Programming
5 Mohannad Al-Kubaisi
Logical Operators
Selection control Statements
Loop control Statements
GO TO statement also called unconditional transfer of control statement is used to
transfer control of execution to another step/statement. . e.g. the statement GOTO
n will transfer control to step/statement n.
C++ Programming
6 Mohannad Al-Kubaisi
Note: We can use keyword INPUT or READ or GET to accept input(s) /value(s) and
keywords PRINT or WRITE or DISPLAY to output the result(s).
OR
C++ Programming
7 Mohannad Al-Kubaisi
C++ Programming
8 Mohannad Al-Kubaisi
C++ Programming
9 Mohannad Al-Kubaisi
C++ Introduction
C++ is a middle-level programming language developed by Bjarne
Stroustrup starting in 1979 at Bell Labs. C++ runs on a variety of platforms,
such as Windows, Mac OS, and the various versions of UNIX.
This C++ tutorial adopts a simple and practical approach to describe the
concepts of C++ for beginners to advanced software engineers.
Why to Learn C++
C++ is necessary for students and working professionals to become a great
Software Engineer. I will list down some of the key advantages of learning
C++:
C++ is very close to hardware, so you get a chance to work at a low
level which gives you lot of control in terms of memory management,
better performance and finally a robust software development.
C++ programming gives you a clear understanding about Object
Oriented Programming. You will understand low-level implementation
of polymorphism when you will implement virtual tables and virtual
table pointers, or dynamic type identification.
C++ is one of the every green programming languages and loved by
millions of software developers. If you are a great C++ programmer,
then you will never sit without work and more importantly, you will get
highly paid for your work.
C++ is the most widely used programming languages in application
and system programming. So you can choose your area of interest of
software development.
C++ really teaches you the difference between compiler, linker and
loader, different data types, storage classes, variable types their
scopes etc.
There are 1000s of good reasons to learn C++ Programming. But one thing
for sure, to learn any programming language, not only C++, you just need
to code, and code and finally code until you become expert.
Applications of C++ Programming
As mentioned before, C++ is one of the most widely used programming
languages. It has it's presence in almost every area of software
development. I'm going to list few of them here:
C++ Programming
10 Mohannad Al-Kubaisi
Application Software Development - C++ programming has been
used in developing almost all the major Operating Systems like
Windows, Mac OSX and Linux. Apart from the operating systems, the
core part of many browsers like Mozilla Firefox and Chrome have
been written using C++. C++ also has been used in developing the
most popular database system called MySQL.
Programming Languages Development - C++ has been used
extensively in developing new programming languages like C#, Java,
JavaScript, Perl, UNIX’s C Shell, PHP and Python, and Verilog etc.
Computation Programming - C++ is the best friends of scientists
because of fast speed and computational efficiencies.
Games Development - C++ is extremely fast which allows
programmers to do procedural programming for CPU intensive
functions and provides greater control over hardware, because of
which it has been widely used in development of gaming engines.
Embedded System - C++ is being heavily used in developing Medical
and Engineering Applications like software’s for MRI machines, highend CAD/CAM systems etc.
This list goes on, there are various areas where software developers are
happily using C++ to provide great software’s. I highly recommend you to
learn C++ and contribute great software’s to the community.
C++ Programming
11 Mohannad Al-Kubaisi
Hello World – First C++ Program
Just to give you a little excitement about C++ programming, we going to give you a
small conventional C++ Hello World program.
C++ is a super set of C programming with additional implementation of object-oriented
concepts.
C++ Program Structure
In this guide, we will write and understand the first program in
C++ programming. We are writing a simple C++ program that prints “Hello
World!” message. Let’s see the program first and then we will discuss each
and every part of it in detail.
#include <iostream>
using namespace std;
// main() is where program execution begins.
int main() {
cout << "Hello World"; // prints Hello World
return 0;
}
Let us look at the various parts of the above program -
The C++ language defines several headers, which contain information
that is either necessary or useful to your program. For this program,
the header <iostream> is needed.
The line using namespace std; tells the compiler to use the std
namespace. Namespaces are a relatively recent addition to C++.
The next line '// main() is where program execution begins.' is a
single-line comment available in C++. Single-line comments begin
with // and stop at the end of the line.
The line int main() is the main function where program execution
begins.
The next line cout << "Hello World"; causes the message "Hello
World" to be displayed on the screen.
The next line return 0; terminates main( )function and causes it to
return the value 0 to the calling process.
C++ Programming
12 Mohannad Al-Kubaisi
Semicolons and Blocks in C++
In C++, the semicolon is a statement terminator. That is, each individual
statement must be ended with a semicolon. It indicates the end of one
logical entity.
For example, following are three different statements -
x = y;
y = y + 1;
add(x, y);
A block is a set of logically connected statements that are surrounded by
opening and closing braces. For example -
{
cout << "Hello World"; // prints Hello World
return 0;
}
C++ does not recognize the end of the line as a terminator. For this reason,
it does not matter where you put a statement in a line. For example -
x = y;
y = y + 1;
add(x, y);
is the same as
x = y; y = y + 1; add(x, y);
C++ Identifiers
A C++ identifier is a name used to identify a variable, function, class,
module, or any other user-defined item. An identifier starts with a letter A to
Z or a to z or an underscore (_) followed by zero or more letters,
underscores, and digits (0 to 9).
C++ does not allow punctuation characters such as @, $, and % within
identifiers. C++ is a case-sensitive programming language.
Thus, Manpower and manpower are two different identifiers in C++.
Here are some examples of acceptable identifiers -
mohd zara abc move_name a_123
myname50 _temp j a23b9 retVal
C++ Programming
13 Mohannad Al-Kubaisi
C++ Keywords
The following list shows the reserved words in C++. These reserved words
may not be used as constant or variable or any other identifier names.
asm | else | new | this |
auto | enum | operator | throw |
bool | explicit | private | true |
break | export | protected | try |
case | extern | public | typedef |
catch | false | register | typeid |
char | float | reinterpret_cast | typename |
class | for | return | union |
const | friend | short | unsigned |
const_cast | goto | signed | using |
continue | if | sizeof | virtual |
default | inline | static | void |
delete | int | static_cast | volatile |
do | long | struct | wchar_t |
double | mutable | switch | while |
dynamic_cast | namespace | template |
Whitespace in C++
A line containing only whitespace, possibly with a comment, is known as a
blank line, and C++ compiler totally ignores it.
Whitespace is the term used in C++ to describe blanks, tabs, newline
characters and comments. Whitespace separates one part of a statement
from another and enables the compiler to identify where one element in a
statement, such as int, ends and the next element begins.
C++ Programming
14 Mohannad Al-Kubaisi
Statement 1
int age;
In the above statement there must be at least one whitespace character (usually a
space) between int and age for the compiler to be able to distinguish them.
Statement 2
fruit = apples + oranges; // Get the total fruit
In the above statement 2, no whitespace characters are necessary between
fruit and =, or between = and apples, although you are free to include some
if you wish for readability purpose.
C++ Programming
15 Mohannad Al-Kubaisi
Comments in C++
Program comments are explanatory statements that you can include in the
C++ code. These comments help anyone reading the source code. All
programming languages allow for some form of comments.
C++ supports single-line and multi-line comments. All characters available
inside any comment are ignored by C++ compiler.
C++ comments start with /* and end with */. For example -
/* This is a comment */
/* C++ comments can also
* span multiple lines
*/
A comment can also start with //, extending to the end of the line. For
example -
#include <iostream>
using namespace std;
main() {
cout << "Hello World"; // prints Hello World
return 0;
}
When the above code is compiled, it will ignore // prints Hello World and
final executable will produce the following result -
Hello World
Within a /* and */ comment, // characters have no special meaning. Within
a // comment, /* and */ have no special meaning. Thus, you can "nest" one
kind of comment within the other kind. For example -
/* Comment out printing of Hello World:
cout << "Hello World"; // prints Hello World
*/
C++ Programming
15 Mohannad Al-Kubaisi
Variables in C++
A variable is a name which is associated with a value that can be changed.
For example when I write int num=20; here variable name is num which is
associated with value 20, int is a data type that represents that this variable
can hold integer values. We will cover the data types in the next tutorial. In
this tutorial, we will discuss about variables.
Syntax of declaring a variable in C++
data_type variable1_name = value1, variable2_name = value2;
For example:
int num1=20, num2=100;
We can also write it like this:
int num1,num2;
num1=20;
num2=100;
Types of variables
Variables can be categorised based on their data type. For example, in the
above example we have seen integer types variables. Following are the
types of variables available in C++.
int: These type of of variables holds integer value.
char: holds character value like ‘c’, ‘F’, ‘B’, ‘p’, ‘q’ etc.
bool: holds boolean value true or false.
double: double-precision floating point value.
float: Single-precision floating point value.
C++ Programming
16 Mohannad Al-Kubaisi
Types of variables based on their scope
Before going further lets discuss what is scope first. When we discussed
the Hello World Program, we have seen the curly braces in the program
like this:
int main {
//Some code
}
Any variable declared inside these curly braces have scope limited within
these curly braces, if you declare a variable in main() function and try to use
that variable outside main() function then you will get compilation error.
Now that we have understood what is scope. Lets move on to the types of
variables based on the scope.
1. Global variable
2. Local variable
Global Variable
A variable declared outside of any function (including main as well) is called
global variable. Global variables have their scope throughout the program,
they can be accessed anywhere in the program, in the main, in the user
defined function, anywhere.
Lets take an example to understand it:
Global variable example
Here we have a global variable myVar, that is declared outside of main. We
have accessed the variable twice in the main() function without any issues.
#include <iostream>
using namespace std;
// This is a global variable
char myVar = 'A';
int main()
C++ Programming
17 Mohannad Al-Kubaisi
{
cout <<"Value of myVar: "<< myVar<<endl;
myVar='Z';
cout <<"Value of myVar: "<< myVar;
return 0;
}
Output:
Value of myVar: A
Value of myVar: Z
Local variable
Local variables are declared inside the braces of any user defined function,
main function, loops or any control statements(if, if-else etc) and have their
scope limited inside those braces.
Local variable example
#include <iostream>
using namespace std;
char myFuncn() {
// This is a local variable
char myVar = 'A';
}
int main()
{
cout <<"Value of myVar: "<< myVar<<endl;
myVar='Z';
cout <<"Value of myVar: "<< myVar;
return 0;
}
Output:
Compile time error, because we are trying to access the
variable myVar outside of its scope. The scope of myVar is limited to the body
of function myFuncn(), inside those braces.
Can global and local variable have same name in
C++?
Lets see an example having same name global and local variable.
#include <iostream>
using namespace std;
C++ Programming
18 Mohannad Al-Kubaisi
// This is a global variable
char myVar = 'A';
char myFuncn() {
// This is a local variable
char myVar = 'B';
return myVar;
}
int main()
{
cout <<"Funcn call: "<< myFuncn()<<endl;
cout <<"Value of myVar: "<< myVar<<endl;
myVar='Z';
cout <<"Funcn call: "<< myFuncn()<<endl;
cout <<"Value of myVar: "<< myVar<<endl;
return 0;
}
Output:
Funcn call: B
Value of myVar: A
Funcn call: B
Value of myVar: Z
As you can see that when I changed the value of myVar in the main function,
it only changed the value of global variable myVar because local
variable myVar scope is limited to the function myFuncn().
C++ Programming
19 Mohannad Al-Kubaisi
C++ Data Types
Data types define the type of data a variable can hold, for example an integer
variable can hold integer data, a character type variable can hold character
data etc.
Data types in C++ are categorised in three groups: Built-in, userdefined and Derived.
Built in data types
char: For characters. Size 1 byte.
char ch = 'A';
int: For integers. Size 2 bytes.
int num = 100;
float: For single precision floating point. Size 4 bytes.
float num = 123.78987;
double: For double precision floating point. Size 8 bytes.
double num = 10098.98899;
C++ Programming
20 Mohannad Al-Kubaisi
bool: For booleans, true or false.
bool b = true;
The following table shows the variable type, how much memory it takes to
store the value in memory, and what is maximum and minimum value which
can be stored in such type of variables.
Type | Typical Bit Width | Typical Range |
char | 1byte | -127 to 127 or 0 to 255 |
unsigned char | 1byte | 0 to 255 |
signed char | 1byte | -127 to 127 |
int | 4bytes | -2147483648 to 2147483647 |
unsigned int | 4bytes | 0 to 4294967295 |
signed int | 4bytes | -2147483648 to 2147483647 |
short int | 2bytes | -32768 to 32767 |
unsigned short int | 2bytes | 0 to 65,535 |
signed short int | 2bytes | -32768 to 32767 |
long int | 8bytes | -2,147,483,648 to 2,147,483,647 |
signed long int | 8bytes | same as long int |
unsigned long int | 8bytes | 0 to 4,294,967,295 |
long long int | 8bytes | -(2^63) to (2^63)-1 |
unsigned long long int | 8bytes | 0 to 18,446,744,073,709,551,615 |
float | 4bytes | |
double | 8bytes | |
long double | 12bytes | |
wchar_t | 2 or 4 bytes | 1 wide character |
The size of variables might be different from those shown in the above table,
depending on the compiler and the computer you are using.
C++ Programming
21 Mohannad Al-Kubaisi
User-defined data types
We have three types of user-defined data types in C++
1. struct
2. union
3. enum
I have covered them in detail in separate tutorials. For now just remember
that these comes under user-defined data types.
Derived data types in C++
We have three types of derived-defined data types in C++
1. Array
2. Function
3. Pointer
They are wide topics of C++ and I have covered them in separate tutorials.
Just follow the tutorials in given sequence and you would be fine.
C++ Programming
22 Mohannad Al-Kubaisi
Variable Scope in C++
A scope is a region of the program and broadly speaking there are three
places, where variables can be declared -
• Inside a function or a block which is called local variables,
• In the definition of function parameters which is called formal
parameters.
• Outside of all functions which is called global variables.
We will learn what is a function and it's parameter in subsequent chapters.
Here let us explain what are local and global variables.
Local Variables
Variables that are declared inside a function or block are local variables.
They can be used only by statements that are inside that function or block
of code. Local variables are not known to functions outside their own.
Following is the example using local variables -
#include <iostream>
using namespace std;
int main () {
// Local variable declaration:
int a, b;
int c;
// actual initialization
a = 10;
b = 20;
c = a + b;
cout << c;
return 0;
}
Global Variables
Global variables are defined outside of all the functions, usually on top of
the program. The global variables will hold their value throughout the lifetime of your program.
C++ Programming
23 Mohannad Al-Kubaisi
A global variable can be accessed by any function. That is, a global variable
is available for use throughout your entire program after its declaration.
Following is the example using global and local variables -
#include <iostream>
using namespace std;
// Global variable declaration:
int g;
int main () {
// Local variable declaration:
int a, b;
// actual initialization
a = 10;
b = 20;
g = a + b;
cout << g;
return 0;
}
A program can have same name for local and global variables but value of
local variable inside a function will take preference. For example -
#include <iostream>
using namespace std;
// Global variable declaration:
int g = 20;
int main () {
// Local variable declaration:
int g = 10;
cout << g;
return 0;
}
When the above code is compiled and executed, it produces the following
result -
10
C++ Programming
24 Mohannad Al-Kubaisi
Initializing Local and Global Variables
When a local variable is defined, it is not initialized by the system, you must
initialize it yourself. Global variables are initialized automatically by the
system when you define them as follows -
Data Type | Initializer |
int | 0 |
char | '\0' |
float | 0 |
double | 0 |
pointer | NULL |
It is a good programming practice to initialize variables properly, otherwise
sometimes program would produce unexpected result.
C++ Programming
25 Mohannad Al-Kubaisi
C++ Constants/Literals
Constants refer to fixed values that the program may not alter and they are
called literals.
Constants can be of any of the basic data types and can be divided into
Integer Numerals, Floating-Point Numerals, Characters, Strings and
Boolean Values.
Again, constants are treated just like regular variables except that their
values cannot be modified after their definition.
Integer Literals
An integer literal can be a decimal, octal, or hexadecimal constant. A prefix
specifies the base or radix: 0x or 0X for hexadecimal, 0 for octal, and nothing
for decimal.
An integer literal can also have a suffix that is a combination of U and L, for
unsigned and long, respectively. The suffix can be uppercase or lowercase
and can be in any order.
Here are some examples of integer literals -
212 // Legal
215u // Legal
0xFeeL // Legal
078 // Illegal: 8 is not an octal digit
032UU // Illegal: cannot repeat a suffix
Following are other examples of various types of Integer literals -
85 // decimal
0213 // octal
0x4b // hexadecimal
30 // int
30u // unsigned int
30l // long
30ul // unsigned long
Floating-point Literals
A floating-point literal has an integer part, a decimal point, a fractional part,
and an exponent part. You can represent floating point literals either in
decimal form or exponential form.
While representing using decimal form, you must include the decimal point,
the exponent, or both and while representing using exponential form, you
C++ Programming
26 Mohannad Al-Kubaisi
must include the integer part, the fractional part, or both. The signed
exponent is introduced by e or E.
Here are some examples of floating-point literals -
3.14159 // Legal
314159E-5L // Legal
510E // Illegal: incomplete exponent
210f // Illegal: no decimal or exponent
.e55 // Illegal: missing integer or fraction
Boolean Literals
There are two Boolean literals and they are part of standard C++ keywords
-
• A value of true representing true.
• A value of false representing false.
You should not consider the value of true equal to 1 and value of false equal
to 0.
Character Literals
Character literals are enclosed in single quotes. If the literal begins with L
(uppercase only), it is a wide character literal (e.g., L'x') and should be
stored in wchar_t type of variable . Otherwise, it is a narrow character literal
(e.g., 'x') and can be stored in a simple variable of char type.
A character literal can be a plain character (e.g., 'x'), an escape sequence
(e.g., '\t'), or a universal character (e.g., '\u02C0').
There are certain characters in C++ when they are preceded by a backslash
they will have special meaning and they are used to represent like newline
(\n) or tab (\t). Here, you have a list of some of such escape sequence codes
Escape sequence | Meaning |
\\ | \ character |
\' | ' character |
\" | " character |
C++ Programming
27 Mohannad Al-Kubaisi
\? | ? character |
\a | Alert or bell |
\b | Backspace |
\f | Form feed |
\n | Newline |
\r | Carriage return |
\t | Horizontal tab |
\v | Vertical tab |
\ooo | Octal number of one to three digits |
\xhh . . . | Hexadecimal number of one or more digits |
Following is the example to show a few escape sequence characters -
#include <iostream>
using namespace std;
int main() {
cout << "Hello\tWorld\n\n";
return 0;
}
When the above code is compiled and executed, it produces the following
result -
Hello World
C++ Programming
28 Mohannad Al-Kubaisi
String Literals
String literals are enclosed in double quotes. A string contains characters
that are similar to character literals: plain characters, escape sequences,
and universal characters.
You can break a long line into multiple lines using string literals and separate
them using whitespaces.
Here are some examples of string literals. All the three forms are identical
strings.
"hello, dear"
"hello, \
dear"
"hello, " "d" "ear"
Defining Constants
There are two simple ways in C++ to define constants -
• Using #define preprocessor.
• Using const keyword.
The #define Preprocessor
Following is the form to use #define preprocessor to define a constant -
#define identifier value
Following example explains it in detail -
#include <iostream>
using namespace std;
#define LENGTH 10
#define WIDTH #define NEWLINE |
5 | '\n' |
int main() {
int area;
area = LENGTH * WIDTH;
cout << area;
cout << NEWLINE;
return 0;
}
C++ Programming
29 Mohannad Al-Kubaisi
When the above code is compiled and executed, it produces the following
result -
50
The const Keyword
You can use const prefix to declare constants with a specific type as follows
const type variable = value;
Following example explains it in detail -
#include <iostream>
using namespace std;
int main() {
const int | LENGTH = 10; | |
const int | WIDTH | = 5; |
const char NEWLINE = int area; |
'\n'; |
area = LENGTH * WIDTH;
cout << area;
cout << NEWLINE;
return 0;
}
When the above code is compiled and executed, it produces the following
result -
50
Note that it is a good programming practice to define constants in
CAPITALS.
C++ Programming
30 Mohannad Al-Kubaisi
Operators in C++
An operator is a symbol that tells the compiler to perform specific
mathematical or logical manipulations. C++ is rich in built-in operators and
provide the following types of operators -
• Arithmetic Operators
• Relational Operators
• Logical Operators
• Bitwise Operators
• Assignment Operators
• Misc Operators
This chapter will examine the arithmetic, relational, logical, bitwise,
assignment and other operators one by one.
Arithmetic Operators
There are following arithmetic operators supported by C++ language -
Assume variable A holds 10 and variable B holds 20, then -
Show Examples
Operator | Description | Example |
+ | Adds two operands | A + B will give 30 |
- | Subtracts second operand from the first | A - B will give -10 |
* | Multiplies both operands | A * B will give 200 |
/ | Divides numerator by de-numerator | B / A will give 2 |
% | Modulus Operator and remainder of after an integer division |
B % A will give 0 |
++ | Increment operator, increases integer value by one |
A++ will give 11 |
-- | Decrement operator, decreases integer value by one |
A-- will give 9 |
C++ Programming
31 Mohannad Al-Kubaisi
Relational Operators
There are following relational operators supported by C++ language
Assume variable A holds 10 and variable B holds 20, then -
Show Examples
Operator | Description | Example |
== | Checks if the values of two operands are equal or not, if yes then condition becomes true. |
(A == B) is not true. |
!= | Checks if the values of two operands are equal or not, if values are not equal then condition becomes true. |
(A != B) is true. |
> | Checks if the value of left operand is greater than the value of right operand, if yes then condition becomes true. |
(A > B) is not true. |
< | Checks if the value of left operand is less than the value of right operand, if yes then condition becomes true. |
(A < B) is true. |
>= | Checks if the value of left operand is greater than or equal to the value of right operand, if yes then condition becomes true. |
(A >= B) is not true. |
<= | Checks if the value of left operand is less than or equal to the value of right operand, if yes then condition becomes true. |
(A <= B) is true. |
Logical Operators
There are following logical operators supported by C++ language.
Assume variable A holds 1 and variable B holds 0, then -
C++ Programming
32 Mohannad Al-Kubaisi
Show Examples
Operator | Description | Example |
&& | Called Logical AND operator. If both the operands are non-zero, then condition becomes true. |
(A && B) is false. |
|| | Called Logical OR Operator. If any of the two operands is non-zero, then condition becomes true. |
(A || B) is true. |
! | Called Logical NOT Operator. Use to reverses the logical state of its operand. If a condition is true, then Logical NOT operator will make false. |
!(A && B) is true. |
Bitwise Operators
Bitwise operator works on bits and perform bit-by-bit operation. The truth
tables for &, |, and ^ are as follows -
p | q | p & q | p | q | p ^ q |
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
1 | 0 | 0 | 1 | 1 |
Assume if A = 60; and B = 13; now in binary format they will be as follows -
A = 0011 1100
B = 0000 1101
C++ Programming
33 Mohannad Al-Kubaisi
-----------------
A&B = 0000 1100
A|B = 0011 1101
A^B = 0011 0001
~A = 1100 0011
The Bitwise operators supported by C++ language are listed in the following
table. Assume variable A holds 60 and variable B holds 13, then -
Show Examples
Operator | Description | Example |
& | Binary AND Operator copies a bit to the result if it exists in both operands. |
(A & B) will give 12 which is 0000 1100 |
| | Binary OR Operator copies a bit if it exists in either operand. |
(A | B) will give 61 which is 0011 1101 |
^ | Binary XOR Operator copies the bit if it is set in one operand but not both. |
(A ^ B) will give 49 which is 0011 0001 |
~ | Binary Ones Complement Operator is unary and has the effect of 'flipping' bits. |
(~A ) will give -61 which is 1100 0011 in 2's complement form due to a signed binary number. |
<< | Binary Left Shift Operator. The left operands value is moved left by the number of bits specified by the right operand. |
A << 2 will give 240 which is 1111 0000 |
>> | Binary Right Shift Operator. The left operands value is moved right by the number of bits specified by the right operand. |
A >> 2 will give 15 which is 0000 1111 |
C++ Programming
34 Mohannad Al-Kubaisi
Assignment Operators
There are following assignment operators supported by C++ language -
Show Examples
Operator | Description | Example |
= | Simple assignment operator, Assigns values from right side operands to left side operand. |
C = A + B will assign value of A + B into C |
+= | Add AND assignment operator, It adds right operand to the left operand and assign the result to left operand. |
C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator, It subtracts right operand from the left operand and assign the result to left operand. |
C -= A is equivalent to C = C - A |
*= | Multiply AND assignment operator, It multiplies right operand with the left operand and assign the result to left operand. |
C *= A is equivalent to C = C * A |
/= | Divide AND assignment operator, It divides left operand with the right operand and assign the result to left operand. |
C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator, It takes modulus using two operands and assign the result to left operand. |
C %= A is equivalent to C = C % A |
<<= | Left shift AND assignment operator. | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator. | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator. | C &= 2 is same as C = C & 2 |
^= | Bitwise exclusive OR and assignment operator. | C ^= 2 is same as C = C ^ 2 |
|= | Bitwise inclusive OR and assignment operator. | C |= 2 is same as C = C | 2 |
C++ Programming
35 Mohannad Al-Kubaisi
Misc Operators
The following table lists some other operators that C++ supports.
Sr.No | Operator & Description |
1 | sizeof sizeof operator returns the size of a variable. For example, sizeof(a), where ‘a’ is integer, and will return 4. |
2 | Condition ? X : Y Conditional operator (?). If Condition is true then it returns value of X otherwise returns value of Y. |
3 | , Comma operator causes a sequence of operations to be performed. The value of the entire comma expression is the value of the last expression of the comma-separated list. |
4 | . (dot) and -> (arrow) Member operators are used to reference individual members of classes, structures, and unions. |
5 | Cast Casting operators convert one data type to another. For example, int(2.2000) would return 2. |
6 | & Pointer operator & returns the address of a variable. For example &a; will give actual address of the variable. |
7 | * Pointer operator * is pointer to a variable. For example *var; will pointer to a variable var. |
Operators Precedence in C++
Operator precedence determines the grouping of terms in an expression.
This affects how an expression is evaluated. Certain operators have higher
precedence than others; for example, the multiplication operator has higher
precedence than the addition operator -
C++ Programming
36 Mohannad Al-Kubaisi
For example x = 7 + 3 * 2; here, x is assigned 13, not 20 because operator
* has higher precedence than +, so it first gets multiplied with 3*2 and then
adds into 7.
Here, operators with the highest precedence appear at the top of the table,
those with the lowest appear at the bottom. Within an expression, higher
precedence operators will be evaluated first.
Show Examples
Category | Operator | Associativity |
Postfix | () [] -> . ++ - - | Left to right |
Unary | + - ! ~ ++ - - (type)* & sizeof | Right to left |
Multiplicative | * / % | Left to right |
Additive | + - | Left to right |
Shift | << >> | Left to right |
Relational | < <= > >= | Left to right |
Equality | == != | Left to right |
Bitwise AND | & | Left to right |
Bitwise XOR | ^ | Left to right |
Bitwise OR | | | Left to right |
Logical AND | && | Left to right |
Logical OR | || | Left to right |
Conditional | ?: | Right to left |
Assignment | = += -= *= /= %=>>= <<= &= ^= |= | Right to left |
Comma | , | Left to right |
C++ Programming
37 Mohannad Al-Kubaisi
C++ decision making statements
Decision making structures require that the programmer specify one or
more conditions to be evaluated or tested by the program, along with a
statement or statements to be executed if the condition is determined to be
true, and optionally, other statements to be executed if the condition is
determined to be false.
Following is the general form of a typical decision making structure found in
most of the programming languages -
C++ programming language provides following types of decision making
statements.
Sr.No | Statement & Description |
1 | if statement An ‘if’ statement consists of a boolean expression followed by one or more statements. |
2 | if...else statement An ‘if’ statement can be followed by an optional ‘else’ statement, which executes when the boolean expression is false. |
3 | switch statement A ‘switch’ statement allows a variable to be tested for equality against a list of values. |
4 | nested if statements You can use one ‘if’ or ‘else if’ statement inside another ‘if’ or ‘else if’ statement(s). |
5 | nested switch statements You can use one ‘switch’ statement inside another ‘switch’ statement(s). |
C++ Programming
38 Mohannad Al-Kubaisi
C++ if statement
An if statement consists of a boolean expression followed by one or more
statements.
Syntax
The syntax of an if statement in C++ is -
if(boolean_expression) {
// statement(s) will execute if the boolean expression is true
}
If the boolean expression evaluates to true, then the block of code inside
the if statement will be executed. If boolean expression evaluates to false,
then the first set of code after the end of the if statement (after the closing
curly brace) will be executed.
Flow Diagram
Example
#include <iostream>
using namespace std;
int main () {
// local variable declaration:
int a = 10;
// check the boolean condition
C++ Programming
39 Mohannad Al-Kubaisi
if( a < 20 ) {
// if condition is true then print the following
cout << "a is less than 20;" << endl;
}
cout << "value of a is : " << a << endl;
return 0;
}
When the above code is compiled and executed, it produces the following
result -
a is less than 20;
value of a is : 10
C++ Programming
40 Mohannad Al-Kubaisi
C++ if...else statement
An if statement can be followed by an optional else statement, which
executes when the boolean expression is false.
Syntax
The syntax of an if...else statement in C++ is -
if(boolean_expression) {
// statement(s) will execute if the boolean expression is true
} else {
// statement(s) will execute if the boolean expression is false
}
If the boolean expression evaluates to true, then the if block of code will be
executed, otherwise else block of code will be executed.
Flow Diagram
Example
Live Demo
#include <iostream>
using namespace std;
int main () {
// local variable declaration:
int a = 100;
C++ Programming
41 Mohannad Al-Kubaisi
// check the boolean condition
if( a < 20 ) {
// if condition is true then print the following
cout << "a is less than 20;" << endl;
} else {
// if condition is false then print the following
cout << "a is not less than 20;" << endl;
}
cout << "value of a is : " << a << endl;
return 0;
}
When the above code is compiled and executed, it produces the following
result -
a is not less than 20;
value of a is : 100
if...else if...else Statement
An if statement can be followed by an optional else if...else statement,
which is very usefull to test various conditions using single if...else if
statement.
When using if , else if , else statements there are few points to keep in mind.
• An if can have zero or one else's and it must come after any else if's.
• An if can have zero to many else if's and they must come before the
else.
• Once an else if succeeds, none of he remaining else if's or else's will
be tested.
Syntax
The syntax of an if...else if...else statement in C++ is -
if(boolean_expression 1) {
// Executes when the boolean expression 1 is true
} else if( boolean_expression 2) {
// Executes when the boolean expression 2 is true
} else if( boolean_expression 3) {
// Executes when the boolean expression 3 is true
} else {
// executes when the none of the above condition is true.
}
C++ Programming
42 Mohannad Al-Kubaisi
Example
#include <iostream>
using namespace std;
int main () {
// local variable declaration:
int a = 100;
// check the boolean condition
if( a == 10 ) {
// if condition is true then print the following
cout << "Value of a is 10" << endl;
} else if( a == 20 ) {
// if else if condition is true
cout << "Value of a is 20" << endl;
} else if( a == 30 ) {
// if else if condition is true
cout << "Value of a is 30" << endl;
} else {
// if none of the conditions is true
cout << "Value of a is not matching" << endl;
}
cout << "Exact value of a is : " << a << endl;
return 0;
}
When the above code is compiled and executed, it produces the following
result -
Value of a is not matching
Exact value of a is : 100
C++ Programming
43 Mohannad Al-Kubaisi
C++ switch statement
A switch statement allows a variable to be tested for equality against a list
of values. Each value is called a case, and the variable being switched on
is checked for each case.
Syntax
The syntax for a switch statement in C++ is as follows -
switch(expression) {
case constant-expression :
statement(s);
break; //optional
case constant-expression :
statement(s);
break; //optional
// you can have any number of case statements.
default : //Optional
statement(s);
}
The following rules apply to a switch statement -
• The expression used in a switch statement must have an integral or
enumerated type, or be of a class type in which the class has a single
conversion function to an integral or enumerated type.
• You can have any number of case statements within a switch. Each
case is followed by the value to be compared to and a colon.
• The constant-expression for a case must be the same data type as
the variable in the switch, and it must be a constant or a literal.
• When the variable being switched on is equal to a case, the statements
following that case will execute until a break statement is reached.
• When a break statement is reached, the switch terminates, and the
flow of control jumps to the next line following the switch statement.
• Not every case needs to contain a break. If no break appears, the flow
of control will fall through to subsequent cases until a break is
reached.
• A switch statement can have an optional default case, which must
appear at the end of the switch. The default case can be used for
C++ Programming
44 Mohannad Al-Kubaisi
performing a task when none of the cases is true. No break is needed
in the default case.
Flow Diagram
Example
#include <iostream>
using namespace std;
int main | () { |
// local variable declaration: | |
char grade = | 'D'; |
switch(grade) {
case | 'A' | : |
cout << "Excellent!" << endl; | ||
break; | ||
case case |
'B' 'C' |
: : |
cout << "Well done" << endl; | ||
break; | ||
case | 'D' | : |
cout << "You passed" << endl;
break;
case 'F' :
cout << "Better try again" << endl;
break;
C++ Programming
45 Mohannad Al-Kubaisi
default :
cout << "Invalid grade" << endl;
}
cout << "Your grade is " << grade << endl;
return 0;
}
This would produce the following result -
You passed
Your grade is D
C++ Programming
46 Mohannad Al-Kubaisi
C++ nested if statements
It is always legal to nest if-else statements, which means you can use one
if or else if statement inside another if or else if statement(s).
Syntax
The syntax for a nested if statement is as follows -
if( boolean_expression 1) {
// Executes when the boolean expression 1 is true
if(boolean_expression 2) {
// Executes when the boolean expression 2 is true
}
}
You can nest else if...else in the similar way as you have
nested if statement.
Example
#include <iostream>
using namespace std;
int main () {
// local variable declaration:
int a = 100;
int b = 200;
// check the boolean condition
if( a == 100 ) {
// if condition is true then check the following
if( b == 200 ) {
// if condition is true then print the following
cout << "Value of a is 100 and b is 200" << endl;
}
}
cout << "Exact value of a is : " << a << endl;
cout << "Exact value of b is : " << b << endl;
return 0;
}
When the above code is compiled and executed, it produces the following
result -
Value of a is 100 and b is 200
Exact value of a is : 100
Exact value of b is : 200
C++ Programming
47 Mohannad Al-Kubaisi
C++ switch statement
A switch statement allows a variable to be tested for equality against a list
of values. Each value is called a case, and the variable being switched on
is checked for each case.
Syntax
The syntax for a switch statement in C++ is as follows -
switch(expression) {
case constant-expression :
statement(s);
break; //optional
case constant-expression :
statement(s);
break; //optional
// you can have any number of case statements.
default : //Optional
statement(s);
}
The following rules apply to a switch statement -
• The expression used in a switch statement must have an integral or
enumerated type, or be of a class type in which the class has a single
conversion function to an integral or enumerated type.
• You can have any number of case statements within a switch. Each
case is followed by the value to be compared to and a colon.
• The constant-expression for a case must be the same data type as
the variable in the switch, and it must be a constant or a literal.
• When the variable being switched on is equal to a case, the statements
following that case will execute until a break statement is reached.
• When a break statement is reached, the switch terminates, and the
flow of control jumps to the next line following the switch statement.
• Not every case needs to contain a break. If no break appears, the flow
of control will fall through to subsequent cases until a break is
reached.
• A switch statement can have an optional default case, which must
appear at the end of the switch. The default case can be used for
performing a task when none of the cases is true. No break is needed
in the default case.
C++ Programming
48 Mohannad Al-Kubaisi
Flow Diagram
Example
#include <iostream>
using namespace std;
int main | () { | |
// local variable declaration: | ||
char grade = | 'D'; | |
switch(grade) { | ||
case | 'A' | : |
cout << "Excellent!" << endl; | ||
break; | ||
case case |
'B' 'C' |
: : |
cout << "Well done" << endl; | ||
break; | ||
case | 'D' | : |
cout << "You passed" << endl;
break;
case | 'F' | : |
cout << "Better try again" << endl; | ||
break; | ||
default | : |
cout << "Invalid grade" << endl;
}
cout << "Your grade is " << grade << endl;
return 0;
}
This would produce the following result -
You passed
Your grade is D
C++ Programming
49 Mohannad Al-Kubaisi
C++ Loop Types
There may be a situation, when you need to execute a block of code several
number of times. In general, statements are executed sequentially: The first
statement in a function is executed first, followed by the second, and so on.
Programming languages provide various control structures that allow for
more complicated execution paths.
A loop statement allows us to execute a statement or group of statements
multiple times and following is the general from of a loop statement in most
of the programming languages -
C++ programming language provides the following type of loops to handle
looping requirements.
Sr.No | Loop Type & Description |
1 | while loop Repeats a statement or group of statements while a given condition is true. It tests the condition before executing the loop body. |
2 | for loop Execute a sequence of statements multiple times and abbreviates the code that manages the loop variable. |
3 | do...while loop Like a ‘while’ statement, except that it tests the condition at the end of the loop body. |
4 | nested loops You can use one or more loop inside any another ‘while’, ‘for’ or ‘do..while’ loop. |
C++ Programming
50 Mohannad Al-Kubaisi
Loop Control Statements
Loop control statements change execution from its normal sequence. When
execution leaves a scope, all automatic objects that were created in that
scope are destroyed.
C++ supports the following control statements.
Sr.No | Control Statement & Description |
1 | break statement Terminates the loop or switch statement and transfers execution to the statement immediately following the loop or switch. |
2 | continue statement Causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating. |
3 | goto statement Transfers control to the labeled statement. Though it is not advised to use goto statement in your program. |
The Infinite Loop
A loop becomes infinite loop if a condition never becomes false. The for loop is
traditionally used for this purpose. Since none of the three expressions that form the ‘for’
loop are required, you can make an endless loop by leaving the conditional expression
empty.
#include <iostream>
using namespace std;
int main () {
for( ; ; ) {
printf("This loop will run forever.\n");
}
return 0;
}
When the conditional expression is absent, it is assumed to be true. You
may have an initialization and increment expression, but C++ programmers
more commonly use the ‘for (;;)’ construct to signify an infinite loop.
NOTE - You can terminate an infinite loop by pressing Ctrl + C keys.
C++ Programming
50 Mohannad Al-Kubaisi
C++ while loop
A while loop statement repeatedly executes a target statement as long as
a given condition is true.
Syntax
The syntax of a while loop in C++ is -
while(condition) {
statement(s);
}
Here, statement(s) may be a single statement or a block of statements.
The condition may be any expression, and true is any non-zero value. The
loop iterates while the condition is true.
When the condition becomes false, program control passes to the line
immediately following the loop.
Flow Diagram
Here, key point of the while loop is that the loop might not ever run. When
the condition is tested and the result is false, the loop body will be skipped
and the first statement after the while loop will be executed.
C++ Programming
51 Mohannad Al-Kubaisi
Example
#include <iostream>
using namespace std;
int main () {
// Local variable declaration:
int a = 10;
// while loop execution
while( a < 20 ) {
cout << "value of a: " << a << endl;
a++;
}
return 0;
}
When the above code is compiled and executed, it produces the following
result -
value of a: 10
value of a: 11
value of a: 12
value of a: 13
value of a: 14
value of a: 15
value of a: 16
value of a: 17
value of a: 18
value of a: 19
C++ Programming
52 Mohannad Al-Kubaisi
C++ for loop
A for loop is a repetition control structure that allows you to efficiently write
a loop that needs to execute a specific number of times.
Syntax
The syntax of a for loop in C++ is -
for ( init; condition; increment ) {
statement(s);
}
Here is the flow of control in a for loop -
• The init step is executed first, and only once. This step allows you to
declare and initialize any loop control variables. You are not required
to put a statement here, as long as a semicolon appears.
• Next, the condition is evaluated. If it is true, the body of the loop is
executed. If it is false, the body of the loop does not execute and flow
of control jumps to the next statement just after the for loop.
• After the body of the for loop executes, the flow of control jumps back
up to the increment statement. This statement can be left blank, as
long as a semicolon appears after the condition.
• The condition is now evaluated again. If it is true, the loop executes
and the process repeats itself (body of loop, then increment step, and
then again condition). After the condition becomes false, the for loop
terminates.
Flow Diagram
C++ Programming
53 Mohannad Al-Kubaisi
Example
#include <iostream>
using namespace std;
int main () {
// for loop execution
for( int a = 10; a < 20; a = a + 1 ) {
cout << "value of a: " << a << endl;
}
return 0;
}
When the above code is compiled and executed, it produces the following
result -
value of a: 10
value of a: 11
value of a: 12
value of a: 13
value of a: 14
value of a: 15
value of a: 16
value of a: 17
value of a: 18
value of a: 19
C++ Programming
54 Mohannad Al-Kubaisi
C++ do...while loop
Unlike for and while loops, which test the loop condition at the top of the
loop, the do...while loop checks its condition at the bottom of the loop.
A do...while loop is similar to a while loop, except that a do...while loop is
guaranteed to execute at least one time.
Syntax
The syntax of a do...while loop in C++ is -
do {
statement(s);
}
while( condition );
Notice that the conditional expression appears at the end of the loop, so the
statement(s) in the loop execute once before the condition is tested.
If the condition is true, the flow of control jumps back up to do, and the
statement(s) in the loop execute again. This process repeats until the given
condition becomes false.
Flow Diagram
C++ Programming
55 Mohannad Al-Kubaisi
Example
#include <iostream>
using namespace std;
int main () {
// Local variable declaration:
int a = 10;
// do loop execution
do {
cout << "value of a: " << a << endl;
a = a + 1;
} while( a < 20 );
return 0;
}
When the above code is compiled and executed, it produces the following
result -
value of a: 10
value of a: 11
value of a: 12
value of a: 13
value of a: 14
value of a: 15
value of a: 16
value of a: 17
value of a: 18
value of a: 19
C++ Programming
56 Mohannad Al-Kubaisi
C++ Functions
A function is a group of statements that together perform a task. Every C++ program has
at least one function, which is main(), and all the most trivial programs can define
additional functions.
You can divide up your code into separate functions. How you divide up your code
among different functions is up to you, but logically the division usually is such that each
function performs a specific task.
A function declaration tells the compiler about a function's name, return type, and
parameters. A function definition provides the actual body of the function.
The C++ standard library provides numerous built-in functions that your program can
call. For example, function strcat() to concatenate two strings, function memcpy() to
copy one memory location to another location and many more functions.
A function is known with various names like a method or a sub-routine or a procedure
etc.
Defining a Function
The general form of a C++ function definition is as follows -
return_type function_name( parameter list ) {
body of the function
}
A C++ function definition consists of a function header and a function body. Here are all
the parts of a function -
• Return Type - A function may return a value. The return_type is the data type
of the value the function returns. Some functions perform the desired operations
without returning a value. In this case, the return_type is the keyword void.
• Function Name - This is the actual name of the function. The function name and
the parameter list together constitute the function signature.
• Parameters - A parameter is like a placeholder. When a function is invoked, you
pass a value to the parameter. This value is referred to as actual parameter or
argument. The parameter list refers to the type, order, and number of the
parameters of a function. Parameters are optional; that is, a function may contain
no parameters.
• Function Body - The function body contains a collection of statements that
define what the function does.
C++ Programming
57 Mohannad Al-Kubaisi
Example
Following is the source code for a function called max(). This function takes two
parameters num1 and num2 and return the biggest of both -
// function returning the max between two numbers
int max(int num1, int num2) {
// local variable declaration
int result;
if (num1 > num2)
result = num1;
else
result = num2;
return result;
}
Function Declarations
A function declaration tells the compiler about a function name and how to call the
function. The actual body of the function can be defined separately.
A function declaration has the following parts -
return_type function_name( parameter list );
For the above defined function max(), following is the function declaration -
int max(int num1, int num2);
Parameter names are not important in function declaration only their type is required, so
following is also valid declaration -
int max(int, int);
Function declaration is required when you define a function in one source file and you
call that function in another file. In such case, you should declare the function at the top
of the file calling the function.
Calling a Function
While creating a C++ function, you give a definition of what the function has to do. To
use a function, you will have to call or invoke that function.
When a program calls a function, program control is transferred to the called function. A
called function performs defined task and when it’s return statement is executed or when
its function-ending closing brace is reached, it returns program control back to the main
program.
To call a function, you simply need to pass the required parameters along with function
name, and if function returns a value, then you can store returned value. For example -
C++ Programming
58 Mohannad Al-Kubaisi
#include <iostream>
using namespace std;
// function declaration
int max(int num1, int num2);
int main () {
// local variable declaration:
int a = 100;
int b = 200;
int ret;
// calling a function to get max value.
ret = max(a, b);
cout << "Max value is : " << ret << endl;
return 0;
}
// function returning the max between two numbers
int max(int num1, int num2) {
// local variable declaration
int result;
if | (num1 > num2) result = num1; |
else
result = num2;
return result;
}
I kept max() function along with main() function and compiled the source code. While
running final executable, it would produce the following result -
Max value is : 200
Function Arguments
If a function is to use arguments, it must declare variables that accept the values of the
arguments. These variables are called the formal parameters of the function.
The formal parameters behave like other local variables inside the function and are
created upon entry into the function and destroyed upon exit.
While calling a function, there are two ways that arguments can be passed to a function
–
C++ Programming
59 Mohannad Al-Kubaisi
Sr.No | Call Type & Description |
1 | Call by Value This method copies the actual value of an argument into the formal parameter of the function. In this case, changes made to the parameter inside the function have no effect on the argument. |
2 | Call by Pointer This method copies the address of an argument into the formal parameter. Inside the function, the address is used to access the actual argument used in the call. This means that changes made to the parameter affect the argument. |
3 | Call by Reference This method copies the reference of an argument into the formal parameter. Inside the function, the reference is used to access the actual argument used in the call. This means that changes made to the parameter affect the argument. |
By default, C++ uses call by value to pass arguments. In general, this means that code
within a function cannot alter the arguments used to call the function and above
mentioned example while calling max() function used the same method.
Default Values for Parameters
When you define a function, you can specify a default value for each of the last
parameters. This value will be used if the corresponding argument is left blank when
calling to the function.
This is done by using the assignment operator and assigning values for the arguments
in the function definition. If a value for that parameter is not passed when the function is
called, the default given value is used, but if a value is specified, this default value is
ignored and the passed value is used instead. Consider the following example -
#include <iostream>
using namespace std;
int sum(int a, int b = 20) {
int result;
result = a + b;
return | (result); |
} | |
int main | () { |
// local variable declaration:
int a = 100;
int b = 200;
int result;
// calling a function to add the values.
result = sum(a, b);
cout << "Total value is :" << result << endl;
// calling a function again as follows.
result = sum(a);
cout << "Total value is :" << result << endl;
return 0;
}
C++ Programming
60 Mohannad Al-Kubaisi
When the above code is compiled and executed, it produces the following result -
Total value is :300
Total value is :120
C++ Programming
61 Mohannad Al-Kubaisi
C++ function call by value
The call by value method of passing arguments to a function copies the actual value of
an argument into the formal parameter of the function. In this case, changes made to the
parameter inside the function have no effect on the argument.
By default, C++ uses call by value to pass arguments. In general, this means that code
within a function cannot alter the arguments used to call the function. Consider the
function swap() definition as follows.
// function definition to swap the values.
void swap(int x, int y) {
int temp;
temp = x; /* save the value of x */
x = y; /* put y into x */
y = temp; /* put x into y */
return;
}
Now, let us call the function swap() by passing actual values as in the following example
#include <iostream>
using namespace std;
// function declaration
void swap(int x, int y);
int main () {
// local variable declaration:
int a = 100;
int b = 200;
cout << "Before swap, value of a :" << a << endl;
cout << "Before swap, value of b :" << b << endl;
// calling a function to swap the values.
swap(a, b);
cout << "After swap, value of a :" << a << endl;
cout << "After swap, value of b :" << b << endl;
return 0;
}
When the above code is put together in a file, compiled and executed, it produces the
following result -
Before swap, value of a :100
Before swap, value of b :200
After swap, value of a :100
After swap, value of b :200
Which shows that there is no change in the values though they had been changed inside
the function.
C++ Programming
62 Mohannad Al-Kubaisi
C++ function call by pointer
The call by pointer method of passing arguments to a function copies the address of
an argument into the formal parameter. Inside the function, the address is used to access
the actual argument used in the call. This means that changes made to the parameter
affect the passed argument.
To pass the value by pointer, argument pointers are passed to the functions just like any
other value. So accordingly you need to declare the function parameters as pointer types
as in the following function swap(), which exchanges the values of the two integer
variables pointed to by its arguments.
// function definition to swap the values.
void swap(int *x, int *y) {
int temp;
temp = *x; /* save the value at address x */
*x = *y; /* put y into x */
*y = temp; /* put x into y */
return;
}
To check the more detail about C++ pointers, kindly check C++ Pointers chapter.
For now, let us call the function swap() by passing values by pointer as in the following
example -
#include <iostream>
using namespace std;
// function declaration
void swap(int *x, int *y);
int main () {
// local variable declaration:
int a = 100;
int b = 200;
cout << "Before swap, value of a :" << a << endl;
cout << "Before swap, value of b :" << b << endl;
/* calling a function to swap the values.
* &a indicates pointer to a ie. address of variable a and
* &b indicates pointer to b ie. address of variable b. */
swap(&a, &b);
cout << "After swap, value of a :" << a << endl;
cout << "After swap, value of b :" << b << endl;
return 0;
}
When the above code is put together in a file, compiled and executed, it produces the
following result -
Before swap, value of a :100
Before swap, value of b :200
After swap, value of a :200
After swap, value of b :100
C++ Programming
63 Mohannad Al-Kubaisi
C++ function call by reference
The call by reference method of passing arguments to a function copies the reference
of an argument into the formal parameter. Inside the function, the reference is used to
access the actual argument used in the call. This means that changes made to the
parameter affect the passed argument.
To pass the value by reference, argument reference is passed to the functions just like
any other value. So accordingly you need to declare the function parameters as
reference types as in the following function swap(), which exchanges the values of the
two integer variables pointed to by its arguments.
// function definition to swap the values.
void swap(int &x, int &y) {
int temp;
temp = x; /* save the value at address x */
x = y; /* put y into x */
y = temp; /* put x into y */
return;
}
For now, let us call the function swap() by passing values by reference as in the following
example -
#include <iostream>
using namespace std;
// function declaration
void swap(int &x, int &y);
int main () {
// local variable declaration:
int a = 100;
int b = 200;
cout << "Before swap, value of a :" << a << endl;
cout << "Before swap, value of b :" << b << endl;
/* calling a function to swap the values using variable
reference.*/
swap(a, b);
cout << "After swap, value of a :" << a << endl;
cout << "After swap, value of b :" << b << endl;
return 0;
}
When the above code is put together in a file, compiled and executed, it produces the
following result -
Before swap, value of a :100
Before swap, value of b :200
After swap, value of a :200
After swap, value of b :100
C++ Programming
64 Mohannad Al-Kubaisi
C++ Recursion
The process in which a function calls itself is known as recursion and the
corresponding function is called the recursive function. The popular example to
understand the recursion is factorial function.
Factorial function: f(n) = n*f(n-1), base condition: if n<=1 then f(n) = 1. Don’t
worry we wil discuss what is base condition and why it is important.
In the following diagram. I have shown that how the factorial function is calling
itself until the function reaches to the base condition.
Lets solve the problem using C++ program.
C++ Programming
65 Mohannad Al-Kubaisi
C++ recursion example: Factorial
#include <iostream>
using namespace std;
//Factorial function
int fact(int n){
/* This is called the base condition, it is
* very important to specify the base condition
* in recursion, otherwise your program will throw
* stack overflow error.
*/
if (n <= 1)
return 1;
else
return n*fact(n-1);
}
int main(){
int num;
cout<<"Enter a number: ";
cin>>num;
cout<<"Factorial of entered number: "<<fact(num);
return 0;
}
Output:
Enter a number: 5
Factorial of entered number: 120
Base condition
In the above program, you can see that I have provided a base condition in the
recursive function. The condition is:
if (n <= 1)
return 1;
The purpose of recursion is to divide the problem into smaller problems till the
base condition is reached. For example in the above factorial program I am
solving the factorial function f(n) by calling a smaller factorial function f(n-1), this
happens repeatedly until the n value reaches base condition(f(1)=1). If you do not
define the base condition in the recursive function then you will get stack
overflow error.
Direct recursion vs indirect recursion
Direct recursion: When function calls itself, it is called direct recursion, the
example we have seen above is a direct recursion example.
C++ Programming
66 Mohannad Al-Kubaisi
Indirect recursion: When function calls another function and that function calls
the calling function, then this is called indirect recursion. For example: function A
calls function B and Function B calls function A.
Indirect Recursion Example in C++
#include <iostream>
using namespace std;
int fa(int);
int fb(int);
int fa(int n){
if(n<=1)
return 1;
else
return n*fb(n-1);
}
int fb(int n){
if(n<=1)
return 1;
else
return n*fa(n-1);
}
int main(){
int num=5;
cout<<fa(num);
return 0;
}
Output:
120
C++ Programming
67 Mohannad Al-Kubaisi
Numbers in C++
Normally, when we work with Numbers, we use primitive data types such as int, short,
long, float and double, etc. The number data types, their possible values and number
ranges have been explained while discussing C++ Data Types.
Defining Numbers in C++
You have already defined numbers in various examples given in previous chapters. Here
is another consolidated example to define various types of numbers in C++ -
#include <iostream>
using namespace std;
int main | () { |
// number definition: | |
short int long float |
s; i; l; f; |
double d;
// number assignments;
s = 10;
i = 1000;
l = 1000000;
f = 230.47;
d = 30949.374;
// number printing;
cout << "short s :" << s << endl;
cout << "int i :" << i << endl;
cout << "long l :" << l << endl;
cout << "float f :" << f << endl;
cout << "double d :" << d << endl;
return 0;
}
When the above code is compiled and executed, it produces the following result -
short s :10
int i :1000
long l :1000000
float f :230.47
double d :30949.4
C++ Programming
68 Mohannad Al-Kubaisi
Math Operations in C++
In addition to the various functions you can create, C++ also includes some useful
functions you can use. These functions are available in standard C and C++ libraries
and called built-in functions. These are functions that can be included in your program
and then use.
C++ has a rich set of mathematical operations, which can be performed on various
numbers. Following table lists down some useful built-in mathematical functions
available in C++.
To utilize these functions you need to include the math header file <cmath>.
Sr.No | Function & Purpose |
1 | double cos(double); This function takes an angle (as a double) and returns the cosine. |
2 | double sin(double); This function takes an angle (as a double) and returns the sine. |
3 | double tan(double); This function takes an angle (as a double) and returns the tangent. |
4 | double log(double); This function takes a number and returns the natural log of that number. |
5 | double pow(double, double); The first is a number you wish to raise and the second is the power you wish to raise it t |
6 | double hypot(double, double); If you pass this function the length of two sides of a right triangle, it will return you the length of the hypotenuse. |
7 | double sqrt(double); You pass this function a number and it gives you the square root. |
8 | int abs(int); This function returns the absolute value of an integer that is passed to it. |
9 | double fabs(double); This function returns the absolute value of any decimal number passed to it. |
10 | double floor(double); Finds the integer which is less than or equal to the argument passed to it. |
C++ Programming
69 Mohannad Al-Kubaisi
Following is a simple example to show few of the mathematical operations -
#include <iostream>
#include <cmath>
using namespace std;
int main | () { |
// number definition: | |
short int long float |
s = 10; i = -1000; l = 100000; f = 230.47; |
double d = 200.374;
// mathematical operations;
cout << "sin(d) :" << sin(d) << endl;
cout << "abs(i) :" << abs(i) << endl;
cout << "floor(d) :" << floor(d) << endl;
cout << "sqrt(f) :" << sqrt(f) << endl;
cout << "pow( d, 2) :" << pow(d, 2) << endl;
return 0;
}
When the above code is compiled and executed, it produces the following result -
sign(d) :-0.634939
abs(i) :1000
floor(d) :200
sqrt(f) :15.1812
pow( d, 2 ) :40149.7
Random Numbers in C++
There are many cases where you will wish to generate a random number. There are
actually two functions you will need to know about random number generation. The first
is rand(), this function will only return a pseudo random number. The way to fix this is to
first call the srand() function.
Following is a simple example to generate few random numbers. This example makes
use of time() function to get the number of seconds on your system time, to randomly
seed the rand() function -
#include <iostream>
#include <ctime>
#include <cstdlib>
using namespace std;
C++ Programming
70 Mohannad Al-Kubaisi
int main () {
int i,j;
// set the seed
srand( (unsigned)time( NULL ) );
/* generate 10 random numbers. */
for( i = 0; i < 10; i++ ) {
// generate actual random number
j = rand();
cout <<" Random Number : " << j << endl;
}
return 0;
}
When the above code is compiled and executed, it produces the following result -
Random Number : 1748144778
Random Number : 630873888
Random Number : 2134540646
Random Number : 219404170
Random Number : 902129458
Random Number : 920445370
Random Number : 1319072661
Random Number : 257938873
Random Number : 1256201101
Random Number : 580322989
C++ Programming
71 Mohannad Al-Kubaisi
C++ Arrays
C++ provides a data structure, the array, which stores a fixed-size sequential collection
of elements of the same type. An array is used to store a collection of data, but it is often
more useful to think of an array as a collection of variables of the same type.
Instead of declaring individual variables, such as number0, number1, ..., and number99,
you declare one array variable such as numbers and use numbers[0], numbers[1], and
..., numbers[99] to represent individual variables. A specific element in an array is
accessed by an index.
All arrays consist of contiguous memory locations. The lowest address corresponds to
the first element and the highest address to the last element.
Declaring Arrays
To declare an array in C++, the programmer specifies the type of the elements and the
number of elements required by an array as follows -
type arrayName [ arraySize ];
This is called a single-dimension array. The arraySize must be an integer constant
greater than zero and type can be any valid C++ data type. For example, to declare a
10-element array called balance of type double, use this statement -
double balance[10];
Initializing Arrays
You can initialize C++ array elements either one by one or using a single statement as
follows -
double balance[5] = {1000.0, 2.0, 3.4, 17.0, 50.0};
The number of values between braces { } can not be larger than the number of elements
that we declare for the array between square brackets [ ]. Following is an example to
assign a single element of the array -
If you omit the size of the array, an array just big enough to hold the initialization is
created. Therefore, if you write -
double balance[] = {1000.0, 2.0, 3.4, 17.0, 50.0};
You will create exactly the same array as you did in the previous example.
balance[4] = 50.0;
The above statement assigns element number 5th in the array a value of 50.0. Array with
4th index will be 5th, i.e., last element because all arrays have 0 as the index of their first
element which is also called base index. Following is the pictorial representaion of the
same array we discussed above -
C++ Programming
72 Mohannad Al-Kubaisi
Accessing Array Elements
An element is accessed by indexing the array name. This is done by placing the index
of the element within square brackets after the name of the array. For example -
double salary = balance[9];
The above statement will take 10th element from the array and assign the value to salary
variable. Following is an example, which will use all the above-mentioned three concepts
viz. declaration, assignment and accessing arrays -
#include <iostream>
using namespace std;
#include <iomanip>
using std::setw;
int main () {
int n[ 10 ]; // n is an array of 10 integers
// initialize elements of array n to 0
for ( int i = 0; i < 10; i++ ) {
n[ i ] = i + 100; // set element at location i to i + 100
}
cout << "Element" << setw( 13 ) << "Value" << endl;
// output each array element's value
for | ( int j = 0; j < 10; j++ ) { cout << setw( 7 )<< j << setw( 13 ) << n[ j ] << endl; |
}
return 0;
}
This program makes use of setw() function to format the output. When the above code
is compiled and executed, it produces the following result -
Element Value
0 100
1 101
2 102
3 103
4 104
5 105
6 106
7 107
8 108
9 109
C++ Programming
73 Mohannad Al-Kubaisi
Arrays in C++
Arrays are important to C++ and should need lots of more detail. There are following few
important concepts, which should be clear to a C++ programmer -
Sr.No | Concept & Description |
1 | Multi-dimensional arrays C++ supports multidimensional arrays. The simplest form of the multidimensional array is the two-dimensional array. |
2 | Pointer to an array You can generate a pointer to the first element of an array by simply specifying the array name, without any index. |
3 | Passing arrays to functions You can pass to the function a pointer to an array by specifying the array's name without an index. |
4 | Return array from functions C++ allows a function to return an array. |
C++ Programming
74 Mohannad Al-Kubaisi
C++ Multi-dimensional Arrays
C++ allows multidimensional arrays. Here is the general form of a multidimensional array
declaration -
type name[size1][size2]...[sizeN];
For example, the following declaration creates a three dimensional 5 . 10 . 4 integer array
int threedim[5][10][4];
Two-Dimensional Arrays
The simplest form of the multidimensional array is the two-dimensional array. A twodimensional array is, in essence, a list of one-dimensional arrays. To declare a twodimensional integer array of size x,y, you would write something as follows -
type arrayName [ x ][ y ];
Where type can be any valid C++ data type and arrayName will be a valid C++
identifier.
A two-dimensional array can be think as a table, which will have x number of rows and
y number of columns. A 2-dimensional array a, which contains three rows and four
columns can be shown as below -
Thus, every element in array a is identified by an element name of the form a[ i ][ j ],
where a is the name of the array, and i and j are the subscripts that uniquely identify
each element in a.
Initializing Two-Dimensional Arrays
Multidimensioned arrays may be initialized by specifying bracketed values for each row.
Following is an array with 3 rows and each row have 4 columns.
int a[3][4] = {
{0, 1, 2, 3} , /* initializers for row indexed by 0 */
{4, 5, 6, 7} , /* initializers for row indexed by 1 */
{8, 9, 10, 11} /* initializers for row indexed by 2 */
};
The nested braces, which indicate the intended row, are optional. The following
initialization is equivalent to previous example -
int a[3][4] = {0,1,2,3,4,5,6,7,8,9,10,11};
C++ Programming
75 Mohannad Al-Kubaisi
Accessing Two-Dimensional Array Elements
An element in 2-dimensional array is accessed by using the subscripts, i.e., row index
and column index of the array. For example -
int val = a[2][3];
The above statement will take 4th element from the 3rd row of the array. You can verify it
in the above digram.
#include <iostream>
using namespace std;
int main | () { |
// an array with 5 rows and 2 columns. | |
int a[5][2] = { | {0,0}, {1,2}, {2,4}, {3,6},{4,8}}; |
// output each array element's value
for | ( int i = 0; i < 5; i++ ) | |
for | ( int j = 0; j < 2; j++ ) | { |
cout << "a[" << i << "][" << j << "]: ";
cout << a[i][j]<< endl;
}
return 0;
}
When the above code is compiled and executed, it produces the following result -
a[0][0]: 0
a[0][1]: 0
a[1][0]: 1
a[1][1]: 2
a[2][0]: 2
a[2][1]: 4
a[3][0]: 3
a[3][1]: 6
a[4][0]: 4
a[4][1]: 8
As explained above, you can have arrays with any number of dimensions, although it is
likely that most of the arrays you create will be of one or two dimensions.
C++ Programming
76 Mohannad Al-Kubaisi
C++ Pointer to an Array
It is most likely that you would not understand this chapter until you go through the
chapter related C++ Pointers.
So assuming you have bit understanding on pointers in C++, let us start: An array name
is a constant pointer to the first element of the array. Therefore, in the declaration -
double balance[50];
balance is a pointer to &balance[0], which is the address of the first element of the array
balance. Thus, the following program fragment assigns p the address of the first element
of balance -
double *p;
double balance[10];
p = balance;
It is legal to use array names as constant pointers, and vice versa. Therefore, *(balance
+ 4) is a legitimate way of accessing the data at balance[4].
Once you store the address of first element in p, you can access array elements using
*p, *(p+1), *(p+2) and so on. Below is the example to show all the concepts discussed
above -
#include <iostream>
using namespace std;
int main () {
// an array with 5 elements.
double balance[5] = {1000.0, 2.0, 3.4, 17.0, 50.0};
double *p;
p = balance;
// output each array element's value
cout << "Array values using pointer " << endl;
for ( int i = 0; i < 5; i++ ) {
cout << "*(p + " << i << ") : ";
cout << *(p + i) << endl;
}
cout << "Array values using balance as address " << endl;
for | ( int i = 0; i < 5; i++ ) { cout << "*(balance + " << i << ") : "; |
cout << *(balance + i) << endl;
}
return 0;
}
C++ Programming
77 Mohannad Al-Kubaisi
When the above code is compiled and executed, it produces the following result -
Array values using pointer
*(p + 0) : 1000
*(p + 1) : 2
*(p + 2) : 3.4
*(p + 3) : 17
*(p + 4) : 50
Array values using balance as address
*(balance + 0) : 1000
*(balance + 1) : 2
*(balance + 2) : 3.4
*(balance + 3) : 17
*(balance + 4) : 50
In the above example, p is a pointer to double which means it can store address of a
variable of double type. Once we have address in p, then *p will give us value available
at the address stored in p, as we have shown in the above example.
C++ Programming
78 Mohannad Al-Kubaisi
C++ Passing Arrays to Functions
C++ does not allow to pass an entire array as an argument to a function. However, You
can pass a pointer to an array by specifying the array's name without an index.
If you want to pass a single-dimension array as an argument in a function, you would
have to declare function formal parameter in one of following three ways and all three
declaration methods produce similar results because each tells the compiler that an
integer pointer is going to be received.
Way-1
Formal parameters as a pointer as follows -
void myFunction(int *param) {
. . .
}
Way-2
Formal parameters as a sized array as follows -
void myFunction(int param[10]) {
. . .
}
Way-3
Formal parameters as an unsized array as follows -
void myFunction(int param[]) {
. . .
}
Now, consider the following function, which will take an array as an argument along with
another argument and based on the passed arguments, it will return average of the
numbers passed through the array as follows -
double getAverage(int arr[], int size) {
int i, sum = 0;
double avg;
for | (i = 0; i < size; ++i) { sum += arr[i]; |
}
avg = double(sum) / size;
return avg;
}
C++ Programming
79 Mohannad Al-Kubaisi
Now, let us call the above function as follows -
#include <iostream>
using namespace std;
// function declaration:
double getAverage(int arr[], int size);
int main () {
// an int array with 5 elements.
int balance[5] = {1000, 2, 3, 17, 50};
double avg;
// pass pointer to the array as an argument.
avg = getAverage( balance, 5 ) ;
// output the returned value
cout << "Average value is: " << avg << endl;
return 0;
}
When the above code is compiled together and executed, it produces the following result
-
Average value is: 214.4
As you can see, the length of the array doesn't matter as far as the function is concerned
because C++ performs no bounds checking for the formal parameters.
Passing multidimensional array to function
In this example we are passing a multidimensional array to the function square which
displays the square of each element.
#include <iostream>
#include <cmath>
using namespace std;
/* This method prints the square of each
* of the elements of multidimensional array
*/
void square(int arr[2][3]){
int temp;
for(int i=0; i<2; i++){
for(int j=0; j<3; j++){
temp = arr[i][j];
cout<<pow(temp, 2)<<endl;
}
}
}
C++ Programming
80 Mohannad Al-Kubaisi
int main(){
int arr[2][3] = {
{1, 2, 3},
{4, 5, 6}
};
square(arr);
return 0;
} 1 4 9
16
25
36
C++ Programming
81 Mohannad Al-Kubaisi
Return Array from Functions in C++
C++ does not allow to return an entire array as an argument to a function. However, you
can return a pointer to an array by specifying the array's name without an index.
If you want to return a single-dimension array from a function, you would have to declare
a function returning a pointer as in the following example -
int * myFunction() {
. . .
}
Second point to remember is that C++ does not advocate to return the address of a local
variable to outside of the function so you would have to define the local variable
as static variable.
Now, consider the following function, which will enter 5 numbers and return them using
an array and call this function as follows -
#include <iostream>
using namespace std;
// function to enter 5 numbers
int * print(){
static int a[5]={1,2,3,4,5};
return a;
}
// main function to call above defined function.
int main()
{
// a pointer to an int.
int * k;
k = print();
for(int j=0; j<5; j++)
cout<< *(k+j)<<"\t";
return 0;
}
When the above code is compiled together and executed, it produces result something
as follows -
1 2 3 4 5
C++ Programming
82 Mohannad Al-Kubaisi
C++ Strings
C++ provides following two types of string representations -
• The C-style character string.
• The string class type introduced with Standard C++.
The C-Style Character String
The C-style character string originated within the C language and continues to be
supported within C++. This string is actually a one-dimensional array of characters which
is terminated by a null character '\0'. Thus a null-terminated string contains the
characters that comprise the string followed by a null.
The following declaration and initialization create a string consisting of the word "Hello".
To hold the null character at the end of the array, the size of the character array
containing the string is one more than the number of characters in the word "Hello."
char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
If you follow the rule of array initialization, then you can write the above statement as
follows -
char greeting[] = "Hello";
Following is the memory presentation of above defined string in C/C++ -
Actually, you do not place the null character at the end of a string constant. The C++
compiler automatically places the '\0' at the end of the string when it initializes the array.
Let us try to print above-mentioned string -
#include <iostream>
using namespace std;
int main () {
char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
cout << "Greeting message: ";
cout << greeting << endl;
return 0;
}
C++ Programming
83 Mohannad Al-Kubaisi
When the above code is compiled and executed, it produces the following result -
Greeting message: Hello
C++ supports a wide range of functions that manipulate null-terminated strings -
Sr.No | Function & Purpose |
1 | strcpy(s1, s2); Copies string s2 into string s1. |
2 | strcat(s1, s2); Concatenates string s2 onto the end of string s1. |
3 | strlen(s1); Returns the length of string s1. |
4 | strcmp(s1, s2); Returns 0 if s1 and s2 are the same; less than 0 if s1<s2; greater than 0 if s1>s2. |
5 | strchr(s1, ch); Returns a pointer to the first occurrence of character ch in string s1. |
6 | strstr(s1, s2); Returns a pointer to the first occurrence of string s2 in string s1. |
Following example makes use of few of the above-mentioned functions -
#include <iostream>
#include <cstring>
using namespace std;
int main () {
char str1[10] = "Hello";
char str2[10] = "World";
char str3[10];
int len ;
// copy str1 into str3
strcpy( str3, str1);
cout << "strcpy( str3, str1) : " << str3 << endl;
// concatenates str1 and str2
strcat( str1, str2);
cout << "strcat( str1, str2): " << str1 << endl;
// total lenghth of str1 after concatenation
len = strlen(str1);
cout << "strlen(str1) : " << len << endl;
return 0;
}
C++ Programming
84 Mohannad Al-Kubaisi
When the above code is compiled and executed, it produces result something as follows
-
strcpy( str3, str1) : Hello
strcat( str1, str2): HelloWorld
strlen(str1) : 10
The String Class in C++
The standard C++ library provides a string class type that supports all the operations
mentioned above, additionally much more functionality. Let us check the following
example -
#include <iostream>
#include <string>
using namespace std;
int main () {
string str1 = "Hello";
string str2 = "World";
string str3;
int len ;
// copy str1 into str3
str3 = str1;
cout << "str3 : " << str3 << endl;
// concatenates str1 and str2
str3 = str1 + str2;
cout << "str1 + str2 : " << str3 << endl;
// total length of str3 after concatenation
len = str3.size();
cout << "str3.size() : " << len << endl;
return 0;
}
When the above code is compiled and executed, it produces result something as follows
-
str3 : Hello
str1 + str2 : HelloWorld
str3.size() : 10
C++ Programming
85 Mohannad Al-Kubaisi
C++ Files and Streams
So far, we have been using the iostream standard library, which
provides cin and cout methods for reading from standard input and writing to standard
output respectively.
This tutorial will teach you how to read and write from a file. This requires another
standard C++ library called fstream, which defines three new data types -
Sr.No | Data Type & Description |
1 | ofstream This data type represents the output file stream and is used to create files and to write information to files. |
2 | ifstream This data type represents the input file stream and is used to read information from files. |
3 | fstream This data type represents the file stream generally, and has the capabilities of both ofstream and ifstream which means it can create files, write information to files, and read information from files. |
To perform file processing in C++, header files <iostream> and <fstream> must be
included in your C++ source file.
Opening a File
A file must be opened before you can read from it or write to it.
Either ofstream or fstream object may be used to open a file for writing. And ifstream
object is used to open a file for reading purpose only.
Following is the standard syntax for open() function, which is a member of fstream,
ifstream, and ofstream objects.
void open(const char *filename, ios::openmode mode);
Here, the first argument specifies the name and location of the file to be opened and the
second argument of the open() member function defines the mode in which the file
should be opened.
Sr.No | Mode Flag & Description |
1 | ios::app Append mode. All output to that file to be appended to the end. |
2 | ios::ate Open a file for output and move the read/write control to the end of the file. |
C++ Programming
86 Mohannad Al-Kubaisi
3 | ios::in Open a file for reading. |
4 | ios::out Open a file for writing. |
5 | ios::trunc If the file already exists, its contents will be truncated before opening the file. |
You can combine two or more of these values by ORing them together. For example if
you want to open a file in write mode and want to truncate it in case that already exists,
following will be the syntax -
ofstream outfile;
outfile.open("file.dat", ios::out | ios::trunc );
Similar way, you can open a file for reading and writing purpose as follows -
fstream afile;
afile.open("file.dat", ios::out | ios::in );
Closing a File
When a C++ program terminates it automatically flushes all the streams, release all the
allocated memory and close all the opened files. But it is always a good practice that a
programmer should close all the opened files before program termination.
Following is the standard syntax for close() function, which is a member of fstream,
ifstream, and ofstream objects.
void close();
Writing to a File
While doing C++ programming, you write information to a file from your program using
the stream insertion operator (<<) just as you use that operator to output information to
the screen. The only difference is that you use an ofstream or fstream object instead of
the cout object.
Reading from a File
You read information from a file into your program using the stream extraction operator
(>>) just as you use that operator to input information from the keyboard. The only
difference is that you use an ifstream or fstream object instead of the cin object.
Read and Write Example
Following is the C++ program which opens a file in reading and writing mode. After
writing information entered by the user to a file named afile.dat, the program reads
information from the file and outputs it onto the screen -
C++ Programming
87 Mohannad Al-Kubaisi
#include <fstream>
#include <iostream>
using namespace std;
int main () {
char data[100];
// open a file in write mode.
ofstream outfile;
outfile.open("afile.dat");
cout << "Writing to the file" << endl;
cout << "Enter your name: ";
cin.getline(data, 100);
// write inputted data into the file.
outfile << data << endl;
cout << "Enter your age: ";
cin >> data;
cin.ignore();
// again write inputted data into the file.
outfile << data << endl;
// close the opened file.
outfile.close();
// open a file in read mode.
ifstream infile;
infile.open("afile.dat");
cout << "Reading from the file" << endl;
infile >> data;
// write the data at the screen.
cout << data << endl;
// again read the data from the file and display it.
infile >> data;
cout << data << endl;
// close the opened file.
infile.close();
return 0;
}
When the above code is compiled and executed, it produces the following sample input
and output -
C++ Programming
88 Mohannad Al-Kubaisi
$./a.out
Writing to the file
Enter your name: Zara
Enter your age: 9
Reading from the file
Zara
9
Above examples make use of additional functions from cin object, like getline() function
to read the line from outside and ignore() function to ignore the extra characters left by
previous read statement.
File Position Pointers
Both istream and ostream provide member functions for repositioning the file-position
pointer. These member functions are seekg ("seek get") for istream and seekp ("seek
put") for ostream.
The argument to seekg and seekp normally is a long integer. A second argument can be
specified to indicate the seek direction. The seek direction can be ios::beg (the default)
for positioning relative to the beginning of a stream, ios::cur for positioning relative to
the current position in a stream or ios::end for positioning relative to the end of a stream.
The file-position pointer is an integer value that specifies the location in the file as a
number of bytes from the file's starting location. Some examples of positioning the "get"
file-position pointer are -
// position to the nth byte of fileObject (assumes ios::beg)
fileObject.seekg( n );
// position n bytes forward in fileObject
fileObject.seekg( n, ios::cur );
// position n bytes back from end of fileObject
fileObject.seekg( n, ios::end );
// position at end of fileObject
fileObject.seekg( 0, ios::end );
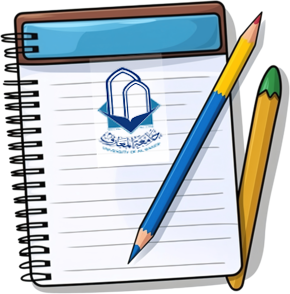